Round, Pipe, and Exporter A Comprehensive Guide
This article aims to provide a comprehensive understanding of the concepts of round, pipe, and exporter. We will explore their individual meanings, their relationships with each other, and how they can be applied in various situations.
**1. Round**
The term round refers to the process of shaping or molding a material into a circular or spherical form. In the context of programming, rounding is often used in mathematical operations to approximate a value to the nearest whole number or a specific number of decimal places. This is commonly used in financial calculations, where precision is essential.
For example, in Python, you can use the `round()` function to round a floating-point number
```python
rounded_value = round(3.14159, 2)
print(rounded_value) Output 3.14
```
**2. Pipe**
A pipe is a conduit or channel that allows the flow of liquids, gases, or data. In programming, a pipe is a communication channel that allows data to be passed between processes or threads. This concept is particularly relevant in Unix-based systems, where pipes are used for inter-process communication (IPC).
Here's an example of using a pipe in a Python script
```python
import subprocess
Run a command and capture its output using a pipe
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE)
output, error = process.communicate()
print(output.decode('utf-8'))
```
**3. Exporter**
An exporter is a person or entity that exports goods or services from one country to another. In programming, an exporter is a component or module that allows data to be exported or shared with other systems or applications. This can be useful for data analysis, reporting, or integrating with other tools.
For instance, in Python, you can use the `csv` module to export data to a CSV file
```python
import csv
data = [['Name', 'Age'], ['Alice', 30], ['Bob', 25]]
with open('output.csv', 'w', newline='') as csvfile
writer = csvcsv', 'w', newline='') as csvfile
writer = csv
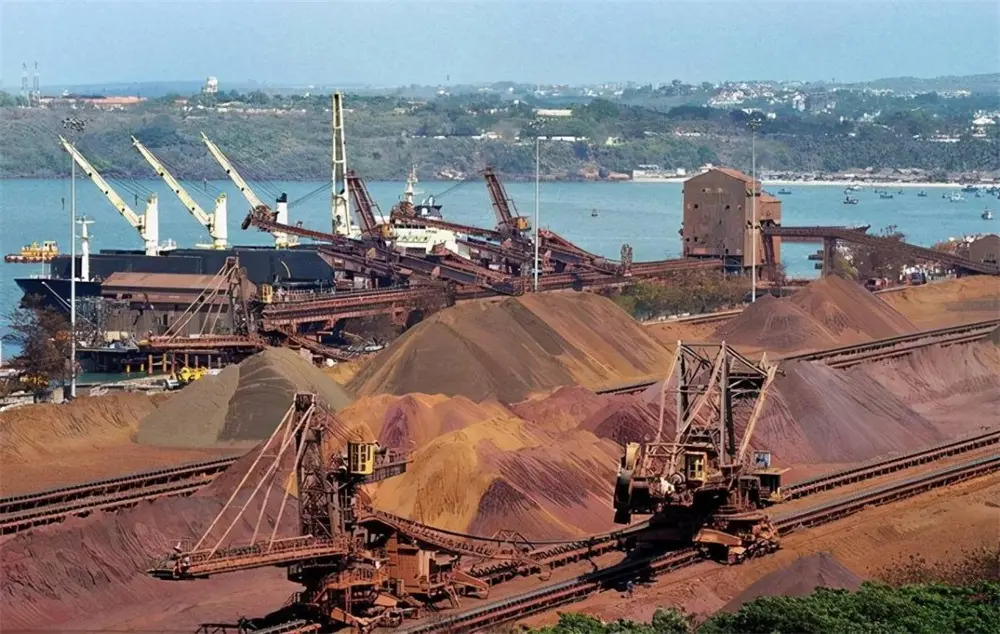
csv', 'w', newline='') as csvfile
writer = csvcsv', 'w', newline='') as csvfile
writer = csv
round pipe exporter.writer(csvfile)
writer.writerows(data)
```
**4. Round, Pipe, and Exporter in Action**
Now let's combine these concepts to create a practical example. Suppose you have a large dataset that you want to process, filter, and export for further analysis. You can use a combination of rounding, pipes, and exporters to achieve this.
```python
import subprocess
import csv
Run a command to fetch data and capture its output using a pipe
process = subprocess.Popen(['cat', 'data.txt'], stdout=subprocess.PIPE)
output, error = process.communicate()
Process the data by rounding values and filtering results
rounded_data = []
for line in output.decode('utf-8').splitlines()
values = [round(float(x), 2) for x in line.split(',')]
if values[1] > 100 Filter only keep values greater than 100
rounded_data.append(values)
Export the filtered and rounded data to a CSV file
with open('filtered_output.csv', 'w', newline='') as csvfile
writer = csv.writer(csvfile)
writer.writerows(rounded_data)
```
In this example, we used a pipe to fetch data from a file, then rounded the values and filtered the results before exporting them to a CSV file. This demonstrates the combined use of rounding, pipes, and exporters in a real-world scenario.
In conclusion, rounding, pipes, and exporters are essential concepts in programming that can be applied in various situations to process, communicate, and share data effectively. Understanding these concepts is crucial for any programmer looking to build robust and efficient applications.